In this document
Downloads
See also
In-app Billing is a Google Play service that provides checkout processing for in-app purchases. To use the service, your application sends a billing request for a specific in-app product. The service then handles all of the checkout details for the transaction, including requesting and validating the form of payment and processing the financial transaction. When the checkout process is complete, the service sends your application the purchase details, such as the order number, the order date and time, and the price paid. At no point does your application have to handle any financial transactions; that role is provided by Google Play's in-app billing service.
In-app Billing Architecture
In-app billing uses an asynchronous message loop to convey billing requests and billing responses between your application and the Google Play server. In practice, your application never directly communicates with the Google Play server (see figure 1). Instead, your application sends billing requests to the Google Play application over interprocess communication (IPC) and receives purchase responses from the Google Play application in the form of asynchronous broadcast intents. Your application does not manage any network connections between itself and the Google Play server or use any special APIs from the Android platform.
Some in-app billing implementations may also use a private remote server to deliver content or validate transactions, but a remote server is not required to implement in-app billing. A remote server can be useful if you are selling digital content that needs to be delivered to a user's device, such as media files or photos. You might also use a remote server to store users' transaction history or perform various in-app billing security tasks, such as signature verification. Although you can handle all security-related tasks in your application, performing those tasks on a remote server is recommended because it helps make your application less vulnerable to security attacks.
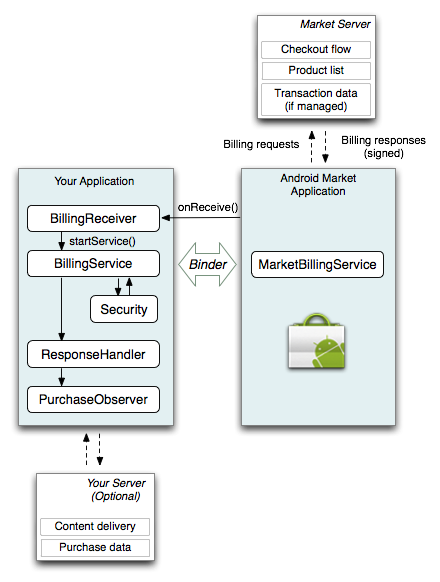
Figure 1. Your application sends and receives billing messages through the Google Play application, which handles all communication with the Google Play server.
A typical in-app billing implementation relies on three components:
- A
Service
(namedBillingService
in the sample application), which processes purchase messages from the application and sends billing requests to the Google Play in-app billing service. - A
BroadcastReceiver
(namedBillingReceiver
in the sample application), which receives all asynchronous billing responses from the Google Play application. - A security component (named
Security
in the sample application), which performs security-related tasks, such as signature verification and nonce generation. For more information about in-app billing security, see Security controls later in this document.
You may also want to incorporate two other components to support in-app billing:
- A response
Handler
(namedResponseHandler
in the sample application), which provides application-specific processing of purchase notifications, errors, and other status messages. - An observer (named
PurchaseObserver
in the sample application), which is responsible for sending callbacks to your application so you can update your user interface with purchase information and status.
In addition to these components, your application must provide a way to store information about users' purchases and some sort of user interface that lets users select items to purchase. You do not need to provide a checkout user interface. When a user initiates an in-app purchase, the Google Play application presents the checkout user interface to your user. When the user completes the checkout process, your application resumes.
In-app Billing Messages
When the user initiates a purchase, your application sends billing messages to Google Play's
in-app billing service (named MarketBillingService
) using simple IPC method calls. The
Google Play application responds to all billing requests synchronously, providing your
application with status notifications and other information. The Google Play application also
responds to some billing requests asynchronously, providing your application with error messages and
detailed transaction information. The following section describes the basic request-response
messaging that takes place between your application and the Google Play application.
In-app billing requests
Your application sends in-app billing requests by invoking a single IPC method
(sendBillingRequest()
), which is exposed by the MarketBillingService
interface. This interface is defined in an Android Interface Definition Language file
(IMarketBillingService.aidl
). You can download this AIDL
file with the in-app billing sample application.
The sendBillingRequest()
method has a single Bundle
parameter.
The Bundle that you deliver must include several key-value pairs that specify various parameters for
the request, such as the type of billing request you are making, the item that is being purchased,
and the application that is making the request. For more information about the Bundle keys that are
sent with a request, see In-app Billing
Service Interface.
One of the most important keys that every request Bundle must have is the
BILLING_REQUEST
key. This key lets you specify the type of billing request you are
making. Google Play's in-app billing service supports the following five types of billing
requests:
CHECK_BILLING_SUPPORTED
This request verifies that the Google Play application supports in-app billing. You usually send this request when your application first starts up. This request is useful if you want to enable or disable certain UI features that are relevant only to in-app billing.
REQUEST_PURCHASE
This request sends a purchase message to the Google Play application and is the foundation of in-app billing. You send this request when a user indicates that he or she wants to purchase an item in your application. Google Play then handles the financial transaction by displaying the checkout user interface.
GET_PURCHASE_INFORMATION
This request retrieves the details of a purchase state change. A purchase changes state when a requested purchase is billed successfully or when a user cancels a transaction during checkout. It can also occur when a previous purchase is refunded. Google Play notifies your application when a purchase changes state, so you only need to send this request when there is transaction information to retrieve.
CONFIRM_NOTIFICATIONS
This request acknowledges that your application received the details of a purchase state change. Google Play sends purchase state change notifications to your application until you confirm that you received them.
RESTORE_TRANSACTIONS
This request retrieves a user's transaction status for managed purchases. You should send this request only when you need to retrieve a user's transaction status, which is usually only when your application is reinstalled or installed for the first time on a device.
In-app Billing Responses
The Google Play application responds to in-app billing requests with both synchronous and
asynchronous responses. The synchronous response is a Bundle
with the following
three keys:
RESPONSE_CODE
This key provides status information and error information about a request.
PURCHASE_INTENT
This key provides a
PendingIntent
, which you use to launch the checkout activity.REQUEST_ID
This key provides you with a request identifier, which you can use to match asynchronous responses with requests.
Some of these keys are not relevant to every request. For more information, see Messaging sequence later in this document.
The asynchronous response messages are sent in the form of individual broadcast intents and include the following:
com.android.vending.billing.RESPONSE_CODE
This response contains a Google Play server response code, and is sent after you make an in-app billing request. A server response code can indicate that a billing request was successfully sent to Google Play or it can indicate that some error occurred during a billing request. This response is not used to report any purchase state changes (such as refund or purchase information). For more information about the response codes that are sent with this response, see Server Response Codes for In-app Billing.
com.android.vending.billing.IN_APP_NOTIFY
This response indicates that a purchase has changed state, which means a purchase succeeded, was canceled, or was refunded. This response contains one or more notification IDs. Each notification ID corresponds to a specific server-side message, and each messages contains information about one or more transactions. After your application receives an
IN_APP_NOTIFY
broadcast intent, you send aGET_PURCHASE_INFORMATION
request with the notification IDs to retrieve message details.com.android.vending.billing.PURCHASE_STATE_CHANGED
This response contains detailed information about one or more transactions. The transaction information is contained in a JSON string. The JSON string is signed and the signature is sent to your application along with the JSON string (unencrypted). To help ensure the security of your in-app billing messages, your application can verify the signature of this JSON string.
The JSON string that is returned with the PURCHASE_STATE_CHANGED
intent provides
your application with the details of one or more billing transactions. An example of this JSON
string is shown below:
{ "nonce" : 1836535032137741465, "orders" : { "notificationId" : "android.test.purchased", "orderId" : "transactionId.android.test.purchased", "packageName" : "com.example.dungeons", "productId" : "android.test.purchased", "developerPayload" : "bGoa+V7g/yqDXvKRqq+JTFn4uQZbPiQJo4pf9RzJ", "purchaseTime" : 1290114783411, "purchaseState" : 0 } }
For more information about the fields in this JSON string, see In-app Billing Broadcast Intents.
Messaging sequence
The messaging sequence for a typical purchase request is shown in figure 2. Request types for
each sendBillingRequest()
method are shown in bold, broadcast intents
are shown in italic. For clarity, figure 2 does not show the RESPONSE_CODE
broadcast intents that are sent for every request.
The basic message sequence for an in-app purchase request is as follows:
- Your application sends a purchase request (
REQUEST_PURCHASE
type), specifying a product ID and other parameters. - The Google Play application sends your application a Bundle with the following keys:
RESPONSE_CODE
,PURCHASE_INTENT
, andREQUEST_ID
. ThePURCHASE_INTENT
key provides aPendingIntent
, which your application uses to start the checkout UI for the given product ID. - Your application launches the pending intent, which launches the checkout UI.
Note: You must launch the pending intent from an activity context and not an application context.
- When the checkout flow finishes (that is, the user successfully purchases the item or cancels
the purchase), Google Play sends your application a notification message (an
IN_APP_NOTIFY
broadcast intent). The notification message includes a notification ID, which references the transaction. - Your application requests the transaction information by sending a
GET_PURCHASE_STATE_CHANGED
request, specifying the notification ID for the transaction. - The Google Play application sends a Bundle with a
RESPONSE_CODE
key and aREQUEST_ID
key. - Google Play sends the transaction information to your application in a
PURCHASE_STATE_CHANGED
broadcast intent. - Your application confirms that you received the transaction information for the given
notification ID by sending a confirmation message (
CONFIRM_NOTIFICATIONS
type), specifying the notification ID for which you received transaction information. - The Google Play application sends your application a Bundle with a
RESPONSE_CODE
key and aREQUEST_ID
key.
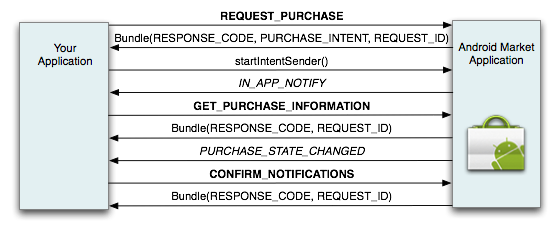
Figure 2. Message sequence for a purchase request.
Keep in mind, you must send a confirmation when you receive transaction information from Google
Play (step 8 in figure 2). If you don't send a confirmation message, Google Play will
continue sending IN_APP_NOTIFY
messages for the transactions you have not
confirmed. As a best practice, you should not send a CONFIRM_NOTIFICATIONS
request for
a purchased item until you have delivered the item to the user. This way, if your application
crashes or something else prevents your application from delivering the product, your application
will still receive an IN_APP_NOTIFY
broadcast intent from Google Play indicating
that you need to deliver the product. Also, as a best practice, your application must be able to
handle IN_APP_NOTIFY
messages that contain multiple orders.
The messaging sequence for a restore transaction request is shown in figure 3. Request types for
each sendBillingRequest()
method are shown in bold, broadcast intents
are shown in italic. For clarity, figure 3 does not show the RESPONSE_CODE
broadcast intents that are sent for every request.
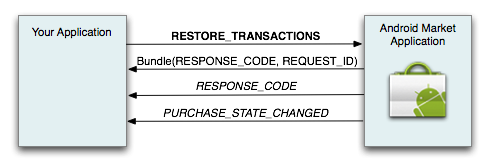
Figure 3. Message sequence for a restore transactions request.
The request triggers three responses. The first is a Bundle
with a
RESPONSE_CODE
key and a REQUEST_ID
key. Next, the Google Play
application sends a RESPONSE_CODE
broadcast intent, which provides status information
or error information about the request. As always, the RESPONSE_CODE
message references
a specific request ID, so you can determine which request a RESPONSE_CODE
message
pertains to.
The RESTORE_TRANSACTIONS
request type also triggers a
PURCHASE_STATE_CHANGED
broadcast intent, which contains the same type of transaction
information that is sent during a purchase request, although you do not need to respond to this
intent with a CONFIRM_NOTIFICATIONS
message.
Note: You should use the RESTORE_TRANSACTIONS
request
type only when your application is installed for the first time on a device or when your
application has been removed from a device and reinstalled.
The messaging sequence for checking whether in-app billing is supported is shown in figure 4. The
request type for the sendBillingRequest()
method is shown in bold.
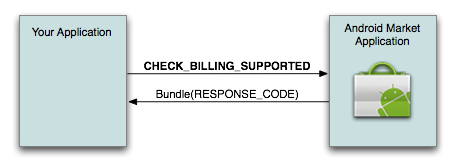
Figure 4. Message sequence for checking whether in-app billing is supported.
The synchronous response for a CHECK_BILLING_SUPPORTED
request provides a Bundle
with a server response code. A RESULT_OK
response code indicates that in-app billing
is supported; a RESULT_BILLING_UNAVAILABLE
response code indicates that in-app billing
is unavailable because the API version you specified is unrecognized or the user is not eligible to
make in-app purchases (for example, the user resides in a country that does not allow in-app
billing). A SERVER_ERROR
can also be returned, indicating that there was a problem with
the Google Play server.
Handling IN_APP_NOTIFY messages
Usually, your application receives an IN_APP_NOTIFY
broadcast intent from Google
Play in response to a REQUEST_PURCHASE
message (see figure 2). The
IN_APP_NOTIFY
broadcast intent informs your application that the state of a requested
purchase has changed. To retrieve the details of that purchase, your application sends a
GET_PURCHASE_INFORMATION
request. Google Play responds with a
PURCHASE_STATE_CHANGED
broadcast intent, which contains the details of the purchase
state change. Your application then sends a CONFIRM_NOTIFICATIONS
message, informing
Google Play that you have received the purchase state change information.
In some special cases, you may receive multiple IN_APP_NOTIFY
messages even though
you have confirmed receipt of the purchase information, or you may receive
IN_APP_NOTIFY
messages for a purchase change even though you never initiated the
purchase. Your application must handle both of these special cases.
Handling multiple IN_APP_NOTIFY messages
When Google Play receives a CONFIRM_NOTIFICATIONS
message for a given
PURCHASE_STATE_CHANGED
message, it usually stops sending IN_APP_NOTIFY
intents for that PURCHASE_STATE_CHANGED
message. Sometimes, however, Google
Play may send repeated IN_APP_NOTIFY
intents for a
PURCHASE_STATE_CHANGED
message even though your application has sent a
CONFIRM_NOTIFICATIONS
message. This can occur if a device loses network connectivity
while you are sending the CONFIRM_NOTIFICATIONS
message. In this case, Google Play
might not receive your CONFIRM_NOTIFICATIONS
message and it could send multiple
IN_APP_NOTIFY
messages until it receives acknowledgement that you received the
transaction message. Therefore, your application must be able to recognize that the subsequent
IN_APP_NOTIFY
messages are for a previously processed transaction. You can do this by
checking the orderID
that's contained in the JSON string because every transaction has
a unique orderId
.
Handling refunds and other unsolicited IN_APP_NOTIFY messages
There are two cases where your application may receive IN_APP_NOTIFY
broadcast
intents even though your application has not sent a REQUEST_PURCHASE
message. Figure 5
shows the messaging sequence for both of these cases. Request types for each
sendBillingRequest()
method are shown in bold, broadcast intents are
shown in italic. For clarity, figure 5 does not show the RESPONSE_CODE
broadcast intents that are sent for every request.
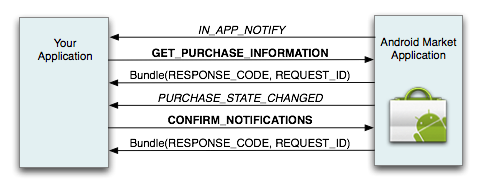
Figure 5. Message sequence for refunds and other unsolicited IN_APP_NOTIFY messages.
In the first case, your application may receive an IN_APP_NOTIFY
broadcast intent
when a user has your application installed on two (or more) devices and the user makes an in-app
purchase from one of the devices. In this case, Google Play sends an IN_APP_NOTIFY
message to the second device, informing the application that there is a purchase state change. Your
application can handle this message the same way it handles the response from an
application-initiated REQUEST_PURCHASE
message, so that ultimately your application
receives a PURCHASE_STATE_CHANGED
broadcast intent message that includes information
about the item that has been purchased. This applies only to items that have their purchase type set
to "managed per user account."
In the second case, your application can receive an IN_APP_NOTIFY
broadcast intent
when Google Play receives a refund notification from Google Checkout. In this case, Google
Play sends an IN_APP_NOTIFY
message to your application. Your application can handle
this message the same way it handles responses from an application-initiated
REQUEST_PURCHASE
message so that ultimately your application receives a
PURCHASE_STATE_CHANGED
message that includes information about the item that has been
refunded. The refund information is included in the JSON string that accompanies the
PURCHASE_STATE_CHANGED
broadcast intent. Also, the purchaseState
field in
the JSON string is set to 2.
Important: You cannot use the Google Checkout API to issue refunds or cancel in-app billing transactions. You must do this manually through your Google Checkout merchant account. However, you can use the Google Checkout API to retrieve order information.
Security Controls
To help ensure the integrity of the transaction information that is sent to your application,
Google Play signs the JSON string that is contained in the PURCHASE_STATE_CHANGED
broadcast intent. Google Play uses the private key that is associated with your publisher account
to create this signature. The publisher site generates an RSA key pair for each publisher account.
You can find the public key portion of this key pair on your account's profile page. It is the same
public key that is used with Google Play licensing.
When Google Play signs a billing response, it includes the signed JSON string (unencrypted) and the signature. When your application receives this signed response you can use the public key portion of your RSA key pair to verify the signature. By performing signature verification you can help detect responses that have been tampered with or that have been spoofed. You can perform this signature verification step in your application; however, if your application connects to a secure remote server then we recommend that you perform the signature verification on that server.
In-app billing also uses nonces (a random number used once) to help verify the integrity of the
purchase information that's returned from Google Play. Your application must generate a nonce and
send it with a GET_PURCHASE_INFORMATION
request and a RESTORE_TRANSACTIONS
request. When Google Play receives the request, it adds the nonce to the JSON string that
contains the transaction information. The JSON string is then signed and returned to your
application. When your application receives the JSON string, you need to verify the nonce as well as
the signature of the JSON string.
For more information about best practices for security and design, see Security and Design.
In-app Billing Requirements and Limitations
Before you get started with in-app billing, be sure to review the following requirements and limitations.
- In-app billing can be implemented only in applications that you publish through Google Play.
- You must have a Google Checkout Merchant account to use Google Play In-app Billing.
- If your device is running Android 3.0, in-app billing requires version 5.0.12 (or higher) of the MyApps application. If your device is running any other version of Android, in-app billing requires version 2.3.4 (or higher) of the Google Play application.
- An application can use in-app billing only if the device is running Android 1.6 (API level 4) or higher.
- You can use in-app billing to sell only digital content. You cannot use in-app billing to sell physical goods, personal services, or anything that requires physical delivery.
- Google Play does not provide any form of content delivery. You are responsible for delivering the digital content that you sell in your applications.
- You cannot implement in-app billing on a device that never connects to the network. To complete in-app purchase requests, a device must be able to access the Google Play server over the network.
For more information about in-app billing requirements, see In-App Billing Availability and Policies.